Background Removal API
Remove the background of any image with 1 API call
Environment
Code
curl -H 'appkey: INSERT_YOUR_API_KEY_HERE' \
-F 'content=@/path/to/file.jpg' \
-f https://pixcut.wondershare.com/openapi/api/v1/matting/removebg \
-o out.png
var request = require('request');
var fs = require('fs');
request.post({
url: 'https://pixcut.wondershare.com/openapi/api/v1/matting/removebg',
formData: {
content: fs.createReadStream('/path/to/file.jpg')
},
headers: {
'appkey': 'INSERT_YOUR_API_KEY_HERE'
},
encoding: null
}, function(error, response, body) {
console.log(response);
});
import requests
response = requests.post(
'https://pixcut.wondershare.com/openapi/api/v1/matting/removebg',
files={'content': open('/path/to/file.jpg', 'rb')},
headers={'appKey': 'INSERT_YOUR_API_KEY_HERE'},
)
with open('out.png', 'wb') as out:
out.write(response.content)
$client = new GuzzleHttp\Client();
$res = $client->post('https://pixcut.wondershare.com/openapi/api/v1/matting/removebg', [
'multipart' => [
[
'name' => 'content',
'contents' => fopen('/path/to/file.jpg', 'r')
]
],
'headers' => [
'APIKEY' => 'INSERT_YOUR_API_KEY_HERE'
]
]);
$fp = fopen("out.png", "wb");
fwrite($fp, $res->getBody());
fclose($fp);
@Autowired
private RestTemplate restTemplate;
File file = new File("/path/to/file.jpg");
byte[] bytesArray = new byte[(int) file.length()];
FileInputStream fis = new FileInputStream(file);
fis.read(bytesArray); //read file into bytes[]
fis.close();
MultipartBodyBuilder builder = new MultipartBodyBuilder();
builder.part("content",bytesArray,MediaType.IMAGE_JPEG);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
headers.add("appkey","INSERT_YOUR_API_KEY_HERE");
HttpEntity<MultiValueMap> request= new HttpEntity<>(builder.build(),headers);
entity = restTemplate.postForEntity("https://pixcut.wondershare.com/openapi/api/v1/matting/removebg", request, Resource.class);
using (var client = new HttpClient())
using (var formData = new MultipartFormDataContent())
{
formData.Headers.Add("appkey", "INSERT_YOUR_API_KEY_HERE");
formData.Add(new ByteArrayContent(File.ReadAllBytes("/path/to/file.jpg")), "content", "file.jpg");
var response = client.PostAsync("https://pixcut.wondershare.com/openapi/api/v1/matting/removebg", formData).Result;
if(response.IsSuccessStatusCode) {
//todo: Handle your logic
} else {
//todo: Handle your logic
}
}
NSURL *fileUrl = [NSBundle.mainBundle URLForResource:@"file" withExtension:@"jpg"];
NSData *data = [NSData dataWithContentsOfURL:fileUrl];
if (!data) {
return;
}
AFHTTPSessionManager *manager =
[[AFHTTPSessionManager alloc] initWithSessionConfiguration:
NSURLSessionConfiguration.defaultSessionConfiguration];https://pixcut.wondershare.com/openapi/api/v1/matting/removebg
manager.responseSerializer = [AFImageResponseSerializer serializer];
[manager.requestSerializer setValue:@"INSERT_YOUR_API_KEY_HERE"
forHTTPHeaderField:@"appkey"];
NSURLSessionDataTask *dataTask = [manager
POST:@"https://pixcut.wondershare.com/openapi/api/v1/matting/removebg"
parameters:nil
constructingBodyWithBlock:^(id _Nonnull formData) {
[formData appendPartWithFileData:data
name:@"content"
fileName:@"file.jpg"
mimeType:@"image/jpeg"];
}
progress:nil
success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
// Handle your logic
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
// Handle your logic
}];
[dataTask resume];
Easy to integrate
Our API is a simple HTTP interface with various options:
- Result images: Image file or JSON-encoded data
- Output resolution: Up to 16 megapixels
- Requires images that have a foreground
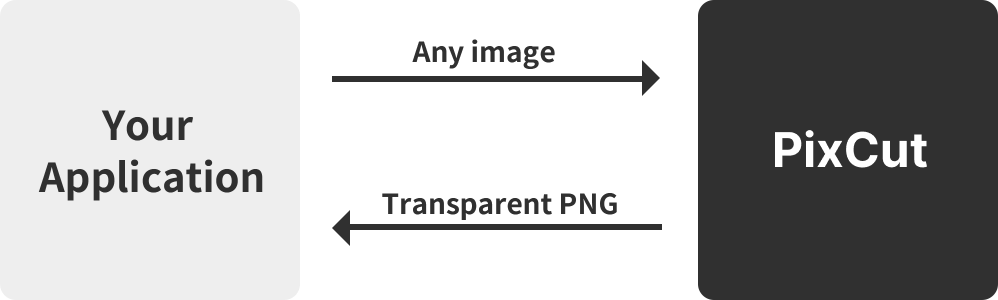
Get started
-
Get your API key.
- Get started quickly with header code examples
- Review the reference docs to adjust any parameters
Request description
HTTP method: POST
Request URL: https://pixcut.wondershare.com/openapi/api/v1/matting/removebg
Header parameters:
parameter | value |
---|---|
Content-Type | multipart/form-data |
appkey | Your Exclusive API Key |
Multipart body parameters:
parameter | file |
---|---|
content | Picture file |
Responses
- Normal response
Output binary format of processed image, content-type is image/png
- Abnormal response
Output application/json format strings
{
"code": 1001,
"data": null,
"msg": 'Request failed',
"time": 1590462453264,
}
Error Code | Description |
---|---|
10001 | Params error |
10002 | Image format error |
30001 | Credit outdate |
30002 | Credit not enough |
40001 | User disable |
40002 | User outdate |
40003 | Appkey error |
40004 | Plantype error |
50001 | Server error |
60004 | File size Fetch error |
60005 | File error |
60006 | File size limit |
60009 | Not matting plan |
60010 | Request error |
60013 | Matting Fail |
60015 | Image Too Large |
Request restrictions
The current API QPS limit is 1 query / second
Product Pricing
You can purchase the number of API calls through online recharge and payment methods. Each successful API call costs 1 credit.
View the price list